Background: Recently I was trying to make a new Video Module in Umbraco that could be added to the grid as a standalone module rather than using the RTE.
To do this I needed to create a custom plugin, I found the little documentation a little confusing as it was generally in the context of a custom property editor rather than a grid editor. Not being that familiar with Umbraco / Angular I have decided to document my findings for anyone in the future so they do not need to jump through the same hoops I did for something that should be relatively simple and quick.
The Task:
Create a custom grid editor that will load an embedded video onto the page and can be added to any column. This video will be embedded via an IFrame using any video provider that supports the embed option. e.g. Youtube / Vimeo
Requirements:
The editor must be re-usable across the site
The user must be able to edit the video from the grid page in Umbraco via the overlay.
The Overlay must persist the current video URL
The Grid must show the video in Umbraco Grid view.
The result page must show the embedded video.
Process:
Step 1: Create a folder in the AppPlugins folder for the Plugin and name it accordingly
Step 2: Create a new html file for the back office view and place in said new folder in AppPlugins folder.
Code Below:
Here the src value ="{{control.value.videoUrl}}" is the value of the video input text box. In a grid editor you need to use "control .value" rather than the "model .value" used in property editors.
Step 3: Create a js file in the same folder in the App_Plugins folder, this will be your controller.
Code below:
angular.module("umbraco").controller("My.VideoModule.Controller", function ($scope, dialogService) {
$scope.openVideoDialog = function () {
// open a custom dialog
dialogService.open({
// set the location of the view
template: "../App_Plugins/VideoDialog/dialog.html",
// pass in data used in dialog
dialogData: $scope.control.value,
// function called when dialog is closed
callback: function (value) {
console.log("callback value url: " + value.videoUrl);
if (value != null && value !== '') {
//$scope.control.value = value.videoUrl;
$scope.control.value = {};
$scope.control.value.videoUrl = value.videoUrl;
/*
$scope.control.value.width = value.width;
$scope.control.value.height = value.height;
*/
}
}
});
};
});
All this controller does is open a dialog window in umbraco, with a custom view specified by the template param:
It specifies the data to pass to the dialog view : $scope.control.value
This value contains all data that is set for the control i.e. if you have multiple fields.
There is also a callback function specified which when the dialog is closed, the data from the dialog is fetched and passed back to the scope data of the control.
We create a new model for the control and set a video Url param of that object model to the videoUrl of the dialog.
Step 4: Create a package.manifest file in the same folder in App_Plugins this informs Umbraco that this new plugin exists and allows you to use it in the back office.
Step 6: Create a Dialog Plugin to be used to configure the Video Url
Again create a new folder in the App_Plugins folder for the dialog. e.g. "VideoDialog"
Step 7: Create a new html view for the dialog and place in the videoDialog folder.
Here we have the form and inputs to submit to the grid editor, also the inputs should be populated if the values have already been set.
The input text with name videoUrl specified and ng-model attribute and a ng-init attribute.
ng-init can be used for setting the initial value if there is any and setting the ng-model value.
ng-model keeps state and validation and registers with the parent form.
Everything else is just a save and close button, which will trigger the callback method in the Grid Editor controller (Not the Dialog Controller).
Step 8: Create a js controller for the Dialog and place in the dialog folder
Here we check if the current video URL is an object i.e. has no value.
if so we set the value of the input equal to an empty string. This is to get around "[object] [object]" being set as the text on load if no value is present.
Otherwise we just set the scope model video equal to the dialogData videoUrl.
Scope Model in the dialog controller is the scope of the dialog view.
Step 9: Create another package.manifest file for the dialog and place in the same dialog folder in the App_Plugins folder, this informs Umbraco of the dialog.
Manifest File:
Step 10: Go to the App_Data/TEMP/ClientDependancy folder and delete the contents, Umbraco can cache controls for the back office, if you do not see your new plugin this could be why, hitting the web.config can also help.
Step 11: Go the the developer section in Umbraco and find the Grid DAta Type and edit the settings of the columns that you want to allow the video module to be used in.
For this example click on 2 column and in the opening dialog select one of the columns, you will be provided with a list of grid editors, your new Video Module should be present here. Tick it and save.
Step 12: Go back to your page and grid tab and select the column and you should be allowed to add a video module.
Video Dialog Opened on clicking link in column:
I hope this helps anyone trying to create their first back office grid editor. It would have greatly helped me if I had it before.
Hi David, no reason at all, it was just a POC for a testing site, I wasn't really thinking of best practice for folder structure. Of course it is up to you as a developer to decide what your folder structure should be like.
You could potentially re-use the dialog with multiple other video modules that you create, in that case it would make sense to separate it out to another folder
It was meant as an informative post for anyone trying to create any type of grid editor not specifically for a video editor.
Although with the embed grid editor you give control of the markup to the content editor, which usually is not the brightest of ideas when rolling out to non technical content editors.
This way you control the markup and make sure that your brand styling is consistent
Hi Dave sorry I though you were taking about the embed HTML option in the Rich text editor. Anyway this post wasn't meant to be about creatin a video editor specifically, it was meant as a guide for creating any grid editor for the first time. I just used a video as an easy example.
Umbraco 7 Create a Custom Grid Editor
Background: Recently I was trying to make a new Video Module in Umbraco that could be added to the grid as a standalone module rather than using the RTE.
To do this I needed to create a custom plugin, I found the little documentation a little confusing as it was generally in the context of a custom property editor rather than a grid editor. Not being that familiar with Umbraco / Angular I have decided to document my findings for anyone in the future so they do not need to jump through the same hoops I did for something that should be relatively simple and quick.
The Task:
Create a custom grid editor that will load an embedded video onto the page and can be added to any column. This video will be embedded via an IFrame using any video provider that supports the embed option. e.g. Youtube / Vimeo
Requirements:
Process:
Step 1: Create a folder in the AppPlugins folder for the Plugin and name it accordingly
Step 2: Create a new html file for the back office view and place in said new folder in AppPlugins folder.
Code Below:
Here the src value ="{{control.value.videoUrl}}" is the value of the video input text box. In a grid editor you need to use "control .value" rather than the "model .value" used in property editors.
Step 3: Create a js file in the same folder in the App_Plugins folder, this will be your controller.
Code below:
All this controller does is open a dialog window in umbraco, with a custom view specified by the template param:
It specifies the data to pass to the dialog view : $scope.control.value
This value contains all data that is set for the control i.e. if you have multiple fields.
There is also a callback function specified which when the dialog is closed, the data from the dialog is fetched and passed back to the scope data of the control.
We create a new model for the control and set a video Url param of that object model to the videoUrl of the dialog.
Step 4: Create a package.manifest file in the same folder in App_Plugins this informs Umbraco that this new plugin exists and allows you to use it in the back office.
This manifest file specifies the alias, name and view to be used by umbraco and also specifies the JS controller.
Step 5: Create a cshtml view for your site to render a custom partial view for the video module, place this in the same plugin folder
Step 6: Create a Dialog Plugin to be used to configure the Video Url
Again create a new folder in the App_Plugins folder for the dialog. e.g. "VideoDialog"
Step 7: Create a new html view for the dialog and place in the videoDialog folder.
Here we have the form and inputs to submit to the grid editor, also the inputs should be populated if the values have already been set.
The input text with name videoUrl specified and ng-model attribute and a ng-init attribute.
ng-init can be used for setting the initial value if there is any and setting the ng-model value.
ng-model keeps state and validation and registers with the parent form.
Everything else is just a save and close button, which will trigger the callback method in the Grid Editor controller (Not the Dialog Controller).
Step 8: Create a js controller for the Dialog and place in the dialog folder
Here we check if the current video URL is an object i.e. has no value. if so we set the value of the input equal to an empty string. This is to get around "[object] [object]" being set as the text on load if no value is present.
Otherwise we just set the scope model video equal to the dialogData videoUrl.
Scope Model in the dialog controller is the scope of the dialog view.
Step 9: Create another package.manifest file for the dialog and place in the same dialog folder in the App_Plugins folder, this informs Umbraco of the dialog.
Manifest File:
Step 10: Go to the App_Data/TEMP/ClientDependancy folder and delete the contents, Umbraco can cache controls for the back office, if you do not see your new plugin this could be why, hitting the web.config can also help.
Step 11: Go the the developer section in Umbraco and find the Grid DAta Type and edit the settings of the columns that you want to allow the video module to be used in.
For this example click on 2 column and in the opening dialog select one of the columns, you will be provided with a list of grid editors, your new Video Module should be present here. Tick it and save.
Step 12: Go back to your page and grid tab and select the column and you should be allowed to add a video module.
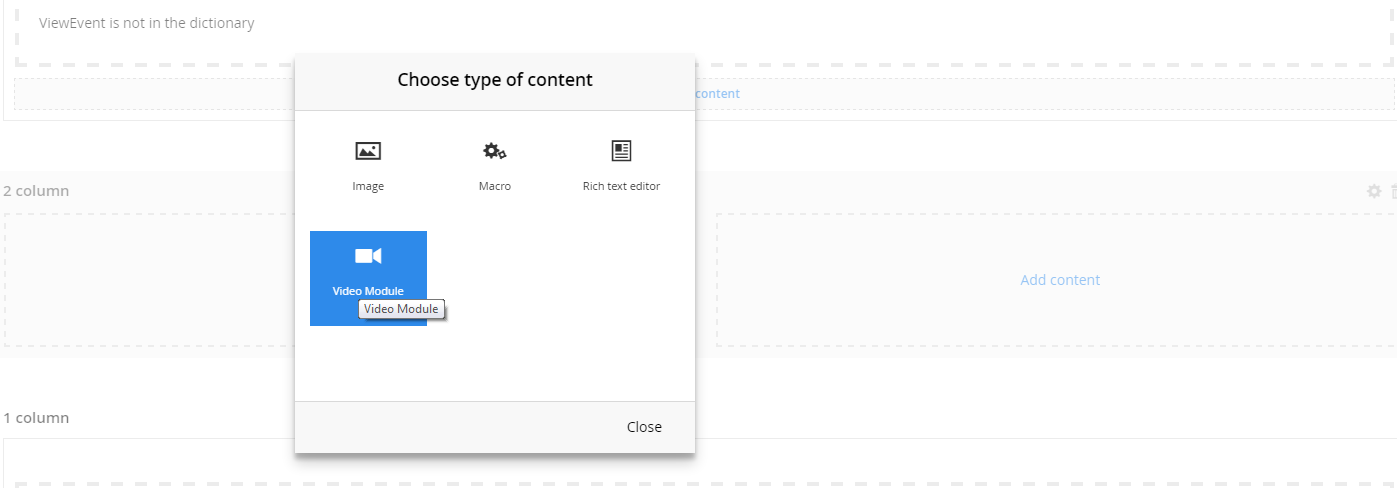
Video Dialog Opened on clicking link in column:
I hope this helps anyone trying to create their first back office grid editor. It would have greatly helped me if I had it before.
Cheers David
Hi David,
was there any special reason why you created the dialog in a seperate folder? Why not put it to the actual grideditor which uses the dialog?
Regards David
Hi David, no reason at all, it was just a POC for a testing site, I wasn't really thinking of best practice for folder structure. Of course it is up to you as a developer to decide what your folder structure should be like.
You could potentially re-use the dialog with multiple other video modules that you create, in that case it would make sense to separate it out to another folder
Hi David,
Why did you create this ? Isn't this possible with Embed grid editor that ships with Umbraco ?
Dave
It was meant as an informative post for anyone trying to create any type of grid editor not specifically for a video editor. Although with the embed grid editor you give control of the markup to the content editor, which usually is not the brightest of ideas when rolling out to non technical content editors.
This way you control the markup and make sure that your brand styling is consistent
How can you give control of the markup to the editor with default embed editor ? Maybe I overlooked that option ?
Dave
Hi Dave sorry I though you were taking about the embed HTML option in the Rich text editor. Anyway this post wasn't meant to be about creatin a video editor specifically, it was meant as a guide for creating any grid editor for the first time. I just used a video as an easy example.
Solution
is working on a reply...