Hello, I extended the ContentService.Saved. The extended code sets the sortOrder to lowest sortOrder - 1, so the node is on the top of content three.
My Code:
public class SortOrderFixerEventHandler : ApplicationEventHandler
{
public SortOrderFixerEventHandler()
{
ContentService.Saved += OnSaved;
}
public static void OnSaved(IContentService sender, SaveEventArgs<IContent> args)
{
var entities = args.SavedEntities.Where(entity => entity.IsNewEntity());
foreach (var entity in entities)
{
var parent = entity.Parent();
if (parent != null)
{
var sortOrder = parent.Children().Min(x => x.SortOrder);
entity.SortOrder = sortOrder - 1;
// save and publish
sender.SaveAndPublishWithStatus(entity);
}
}
}
The logic is working, the only problem I have is the "save and publish" at the end.
When I save a content, inside the History tab it creates two audit items which are the same.
I tried removing the sender.SaveAndPublishWithStatus(entity); line but without this my logic didn't get applied. however when removing the line it only created one audit item so i am sure the problem is with the save and publish line.
How can I save my changes but only create one audit item in the history tab?
Duplicate "Save and Publish" in history tab
Hello, I extended the ContentService.Saved. The extended code sets the sortOrder to lowest sortOrder - 1, so the node is on the top of content three.
My Code:
The logic is working, the only problem I have is the "save and publish" at the end.
When I save a content, inside the History tab it creates two audit items which are the same.
I tried removing the
sender.SaveAndPublishWithStatus(entity);
line but without this my logic didn't get applied. however when removing the line it only created one audit item so i am sure the problem is with the save and publish line.How can I save my changes but only create one audit item in the history tab?
Gif of the issue with the code from above: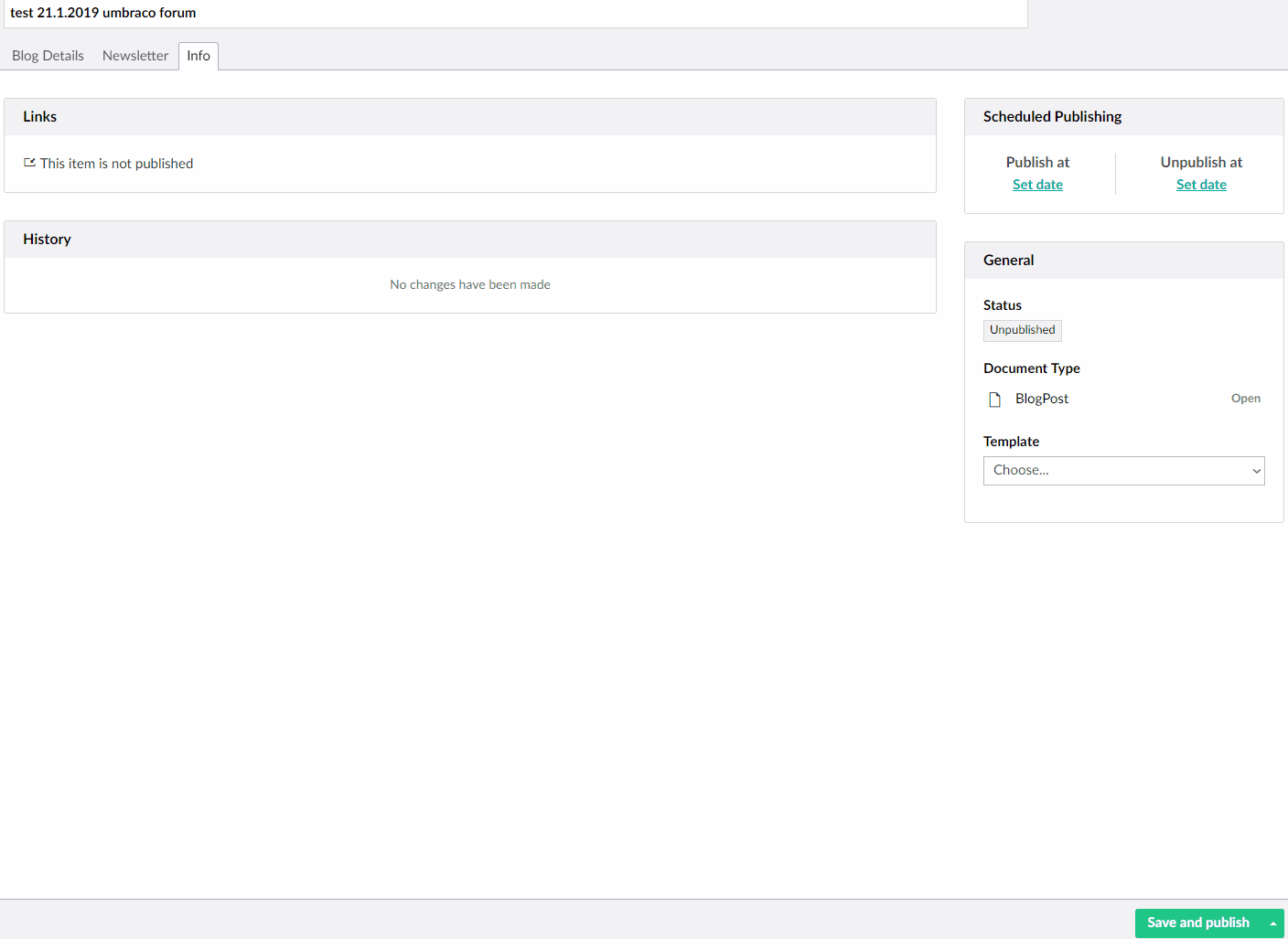
The reason there are two audit trail records is because you're hitting the "Published" method twice.
Once on the initial button click of "Save and Publish", and again when you re-call it in your "Saved" method.
To avoid this, you could perform your logic on the "Saving" method, like so:
Hopefully, this resolves things.
is working on a reply...