var contentTypeBaseServiceProvider = Current.Services.ContentTypeBaseServices;
IMedia image = _umbMediaService.InsertImage(model.Logo, model.MediaFolderId, contentTypeBaseServiceProvider);
Here are the using statements. I am sure not all are necessary. I am sure your visual studios intellisesne will tell you if one of them is not required.
using Umbraco.Core.Composing;
using Website.Core.Helpers;
using Website.Core.Models;
using Website.Core.Models.EntityModels;
using Website.Core.Services;
I can confirm this code works. I literally just re-tested this on a project before posting.
I was struggling with this while trying to write a plugin that would allow fetching images from a web url and storing them in a folder. I ended up solving it in Umbraco 8.10.1 using the following code:
public bool SaveImageByUrl(string url, IMedia mediaFolder, string fileName)
{
try
{
IMedia media = Services.MediaService.CreateMediaWithIdentity(fileName, mediaFolder, Constants.Conventions.MediaTypes.Image);
WebClient wc = new WebClient();
using (MemoryStream memoryStream = new MemoryStream(wc.DownloadData(url)))
{
media.SetValue(_contentTypeBaseServiceProvider, Constants.Conventions.Media.File, fileName, memoryStream);
media.SetValue("alternativeText", "demo");
Services.MediaService.Save(media);
}
return true;
}
catch (System.Exception ex)
{
Logger.Error(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType, "Error ocurred while importing image.", ex);
throw ex;
}
}
Note that the _contentTypeBaseServiceProvider has to be imported via dependency injection in the constructor of your controller.
If you need to create the stream from a local file on disk, you can instead use File.OpenRead("path-to-your-file").
These are my import statements:
using Umbraco.Core;
using Umbraco.Core.Services;
using Umbraco.Web.WebApi;
using Web.Models;
using Constants = Umbraco.Core.Constants;
Upload Image to media
Hi Guys, I am trying to upload a Image, but I get this error.
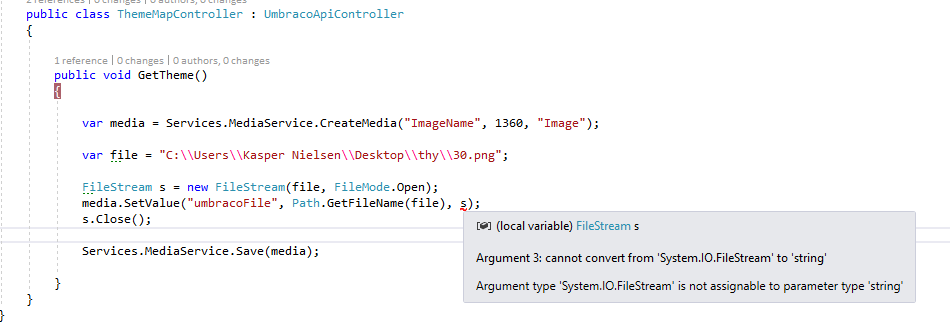
You could use a method like this:
And call it like this:
And you should be able to inject the IContentTypeBaseServiceProvider into your controller instead of using Current. But see if this works first.
This does not work, for starters there isn't a overload on IMedia.SetValue() that takes a ContentTypeBaseServiceProvider.
I've tried with the following:
But this hasn't worked either. I have no idea what to do with the file in order for it to be correctly added to the Media library.
Take a look at this post.
You need to reference Umbraco.Core - the setvalue method that takes ContentTypeBaseServiceProvider resides in Umbraco.Core.ContentExtensions
Hi,
This is how I do it.
And this is how I call the method.
Here are the using statements. I am sure not all are necessary. I am sure your visual studios intellisesne will tell you if one of them is not required.
I can confirm this code works. I literally just re-tested this on a project before posting.
Regards
David
I was struggling with this while trying to write a plugin that would allow fetching images from a web url and storing them in a folder. I ended up solving it in Umbraco 8.10.1 using the following code:
Note that the _contentTypeBaseServiceProvider has to be imported via dependency injection in the constructor of your controller.
If you need to create the stream from a local file on disk, you can instead use File.OpenRead("path-to-your-file").
These are my import statements:
is working on a reply...