I'm trying to upload files via angularJs in the backoffice, and have already tried the UmbracoAuthorizedApiController, UmbracoApiController, and even the SurfaceController (to make sure I'm not going crazy).
And yet, it keeps submitting null files. I attempted several different tutorials online, but nothing works. I'm sure it's something trivial, maybe someone can see it and point me in the right direction, please?
If you define your action as UploadFile(object data) does data then have a value of Object rather than null? If so then it is probably the data mapping that is incorrect resulting in data being null as data is not an IFormFile
Have you tried IFormFileCollection instead of IFormFile or even just IFormCollection? Form posts are normally a collection even if it is only one file
Yes, it does return an Object. and IFormFileCollection returns an empty list
and so does IFormCollection
I agree that I'm probably mapping the model with the wrong type, but what type should be used, then? Every example and tutorial I've found uses IFormFile (comes null), or even IList<IFormFile> (comes empty) and those are not working.
I've never done it myself so not sure what else to suggest. If I have time later I'll have a twiddle and see if I can work it out, if nobody else comes up with an answer
I am trying to do the same thing in Umbraco 10 but rather than having a separate back office section I am looking to override the existing media upload process essentially bypassing the upload process with a form:
I have implemented this on v7 and looking to port onto v10 but the angular script isn't even getting hit am I missing a step.
Will have a look but was looking to use HTTP interceptor which presented the user with the modal shown above and then pass additional info into the media fields based on their selection.
Okay new idea could I create an angular form within the interceptor that reads the user values as well as the file details then pass this to the notification handler to do the rest?
Uploading files via angularJs in Backoffice
I'm trying to upload files via angularJs in the backoffice, and have already tried the UmbracoAuthorizedApiController, UmbracoApiController, and even the SurfaceController (to make sure I'm not going crazy).
The C# Controller has this method:
and the angular controller is performing this
and the angularJs resource file has this post
And yet, it keeps submitting null files. I attempted several different tutorials online, but nothing works. I'm sure it's something trivial, maybe someone can see it and point me in the right direction, please?
If you define your action as
UploadFile(object data)
does data then have a value of Object rather than null? If so then it is probably the data mapping that is incorrect resulting in data being null as data is not an IFormFileHave you tried IFormFileCollection instead of IFormFile or even just IFormCollection? Form posts are normally a collection even if it is only one file
Thanks Huw,
Yes, it does return an Object. and IFormFileCollection returns an empty list
and so does IFormCollection
I agree that I'm probably mapping the model with the wrong type, but what type should be used, then? Every example and tutorial I've found uses IFormFile (comes null), or even
IList<IFormFile>
(comes empty) and those are not working.What am I missing?
I've never done it myself so not sure what else to suggest. If I have time later I'll have a twiddle and see if I can work it out, if nobody else comes up with an answer
I cam across this posted by Kevin, although it is for version 8, I believe it is still valid in 9+
https://github.com/KevinJump/DoStuffWithUmbraco/tree/master/Src/DoStuff.Core/FileUpload
Thank you Huw,
I got it to work like this..
angularjs controller
view.html
ImportAuthorizedApiController C#
Also had to update the
maxAllowedContentLength
to allow large files insideapplicationhost.config
(or web.config)It turns out that I needed to set a local variable for
Request.Form.Files
Thank you for your help
Can I ask what class
_extensions
is ?I don't think so, as v8 uses .NET Framework, and 9 uses .NET Core, some code in the Controller that receives the file.
Carlos' answer below is correct
I am trying to do the same thing in Umbraco 10 but rather than having a separate back office section I am looking to override the existing media upload process essentially bypassing the upload process with a form: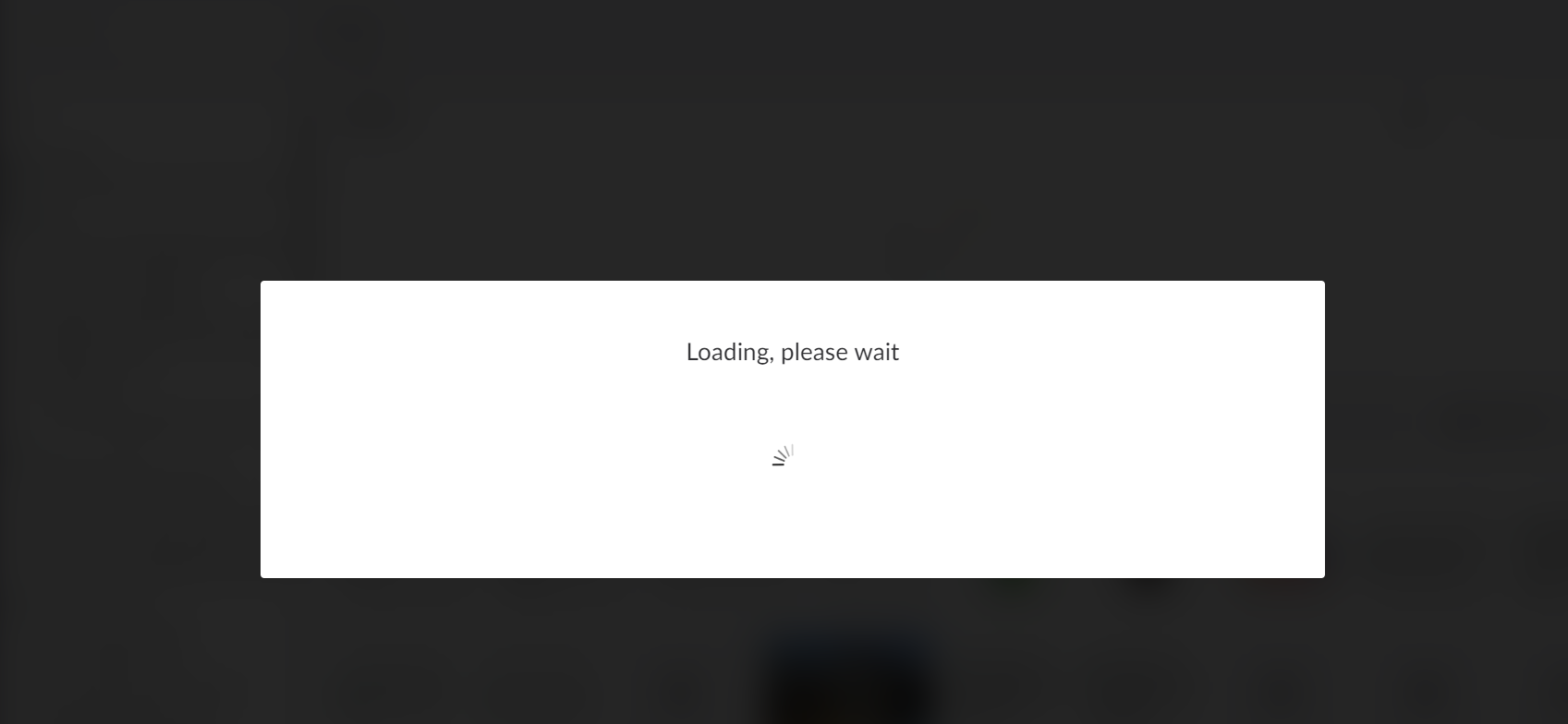
I have implemented this on v7 and looking to port onto v10 but the angular script isn't even getting hit am I missing a step.
Hi Andrew,
Have you tried the notification service?
https://docs.umbraco.com/umbraco-cms/reference/notifications/mediaservice-notifications
I'm not sure how else you'd be able to tap into the existing controls.
Will have a look but was looking to use HTTP interceptor which presented the user with the modal shown above and then pass additional info into the media fields based on their selection.
Okay new idea could I create an angular form within the interceptor that reads the user values as well as the file details then pass this to the notification handler to do the rest?
is working on a reply...